Web3.js: Interacting with AIOZRC-20 Token Smart Contract
In dApps development in the blockchain world, there are two main components to build:
- Smart Contract: writing smart contract by Solidity programming language and deploy into blockchain with developer tools such as Hardhat, Remix, Truffle
- Developing websites or clients that interact with the blockchain: writing code that reads and writes data from the blockchain with smart contracts: Web3.js, Ethers.js
Web3.js is a collection of libraries that allows you to develop websites or clients that interact with the EVM blockchains. Web3.js can be used on frontends and backends to read data from the blockchain or make transactions and broadcast to the blockchain.
In AIOZRC-20 Token Tutorial, we deployed AIOZRC-20 Token Smart Contract. This tutorial will use Web3.js to interact with deployed smart contracts.
Install Web3.js
npm install web3
Query AIOZ Balance
Create web3.js
script as follow:
const Web3 = require('web3')
// AIOZ Testnet RPC
const rpcURL = "https://eth-ds.testnet.aioz.network"
const web3 = new Web3(rpcURL)
// Query balance AIOZ of this address
const address = "0x2993D403b9FBFCec3d2dB34a9BFCF11FFaEe062d"
web3.eth.getBalance(address, (err, wei) => {
balance = web3.utils.fromWei(wei, 'ether')
console.log("AIOZ Balance: " + balance)
})
Output:
$ node web3.js
AIOZ Balance: 0.0946035525
Query Balance from AIOZRC-20 Token Smart Contract
const Web3 = require('web3')
// AIOZ Testnet RPC
const rpcURL = "https://eth-ds.testnet.aioz.network"
const web3 = new Web3(rpcURL)
// Query MyToken balance of this address
const address = "0x2993D403b9FBFCec3d2dB34a9BFCF11FFaEe062d"
// After compilation process, Smart Contract ABI (Abstract Binary Interface) is created, copy it from Remix Solidity Compiler.
const abi = [{"inputs":[],"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"owner","type":"address"},{"indexed":true,"internalType":"address","name":"spender","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Approval","type":"event"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"approve","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"subtractedValue","type":"uint256"}],"name":"decreaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"addedValue","type":"uint256"}],"name":"increaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"to","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transfer","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"from","type":"address"},{"indexed":true,"internalType":"address","name":"to","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Transfer","type":"event"},{"inputs":[{"internalType":"address","name":"from","type":"address"},{"internalType":"address","name":"to","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transferFrom","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"owner","type":"address"},{"internalType":"address","name":"spender","type":"address"}],"name":"allowance","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"account","type":"address"}],"name":"balanceOf","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"decimals","outputs":[{"internalType":"uint8","name":"","type":"uint8"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"name","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"symbol","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"totalSupply","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"}]
// MyToken Smart Contract Address
const contractAddress = "0xA6B996f9f777093785d20005122dC34bd1C1BbBf"
// Init Contract Instance from Web3
const contract = new web3.eth.Contract(abi, contractAddress)
// Call function of Smart Contract to read balance
contract.methods.balanceOf(address).call((err, result) => {
var balanceToken = web3.utils.fromWei(result, 'ether')
console.log("MyToken Balance: " + balanceToken)
})
Output:
$ node web3.js
MyToken Balance: 1000
Send Transaction: Transfer AIOZRC-20 Token
Install Package HDWalletProvider
npm i @truffle/hdwallet-provider --save
Import Account Into Web3.js With HDWalletProvider
const HDWalletProvider = require("@truffle/hdwallet-provider");
const Web3 = require('web3')
const rpcURL = "https://eth-ds.testnet.aioz.network"
const privateKey = "PRIVATE KEY OF ACCOUNT SENDER"
// Import account into Web3 instance to sign transactions
const provider = new HDWalletProvider(privateKey, rpcURL);
const web3 = new Web3(provider)
web3.eth.getAccounts().then(accounts => {
console.log(accounts[0])
});
Send AIOZRC-20 Token With Web3.js
const abi = [{"inputs":[],"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"owner","type":"address"},{"indexed":true,"internalType":"address","name":"spender","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Approval","type":"event"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"approve","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"subtractedValue","type":"uint256"}],"name":"decreaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"addedValue","type":"uint256"}],"name":"increaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"to","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transfer","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"from","type":"address"},{"indexed":true,"internalType":"address","name":"to","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Transfer","type":"event"},{"inputs":[{"internalType":"address","name":"from","type":"address"},{"internalType":"address","name":"to","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transferFrom","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"owner","type":"address"},{"internalType":"address","name":"spender","type":"address"}],"name":"allowance","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"account","type":"address"}],"name":"balanceOf","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"decimals","outputs":[{"internalType":"uint8","name":"","type":"uint8"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"name","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"symbol","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"totalSupply","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"}]
const contractAddress = "0xA6B996f9f777093785d20005122dC34bd1C1BbBf"
const contract = new web3.eth.Contract(abi, contractAddress)
const fromAddress = "0x2993D403b9FBFCec3d2dB34a9BFCF11FFaEe062d"
const toAddress = "0xCd43Fc9aABf694027B31081bAA8F872f2CC125F9"
let amountToken = 5
const tx = {
from: fromAddress,
to: contractAddress,
value: "0x0",
gasLimit: web3.utils.toHex(50000),
gasPrice: web3.utils.toHex(web3.utils.toWei('10', 'gwei')),
data: contract.methods.transfer(toAddress, web3.utils.toWei(amountToken.toString(), 'ether')).encodeABI()
};
web3.eth.accounts.signTransaction(tx, privateKey).then((signedTx) => {
const sentTx = web3.eth.sendSignedTransaction(signedTx.raw || signedTx.rawTransaction);
sentTx.on("receipt", receipt => {
console.log(receipt)
});
sentTx.on("error", err => {
console.log(err)
});
}).catch((err) => {
console.log(err)
});
Output:
$ node web3.js
{
blockHash: '0x859468f81543769b585021f69481eae568643d694e0a938a4fa43c09a302d431',
blockNumber: 2199836,
contractAddress: null,
cumulativeGasUsed: 35293,
from: '0x2993d403b9fbfcec3d2db34a9bfcf11ffaee062d',
gasUsed: 35293,
logs: [
{
address: '0xA6B996f9f777093785d20005122dC34bd1C1BbBf',
topics: [Array],
data: '0x0000000000000000000000000000000000000000000000004563918244f40000',
blockNumber: 2199836,
transactionHash: '0x68b19d52a6be96df2fede99387d501ce5281c9953cca98b287be18844e2a1041',
transactionIndex: 0,
blockHash: '0x859468f81543769b585021f69481eae568643d694e0a938a4fa43c09a302d431',
logIndex: 0,
removed: false,
id: 'log_14d28a72'
}
],
logsBloom: '0x00000000000000000000000000000020000000000000000000000000000000100000000000000000000000000002000000000000000000000800000000000000000000000000000000000008000000000000000400000000000000000000000000000000000000000000000000000020000080000000000000000010000000000001000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000002000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000040000000000000000',
status: true,
to: '0xa6b996f9f777093785d20005122dc34bd1c1bbbf',
transactionHash: '0x68b19d52a6be96df2fede99387d501ce5281c9953cca98b287be18844e2a1041',
transactionIndex: 0,
type: '0x0'
}
Check your TX details from AIOZ Blockchain Explorer Testnet (opens in a new tab)
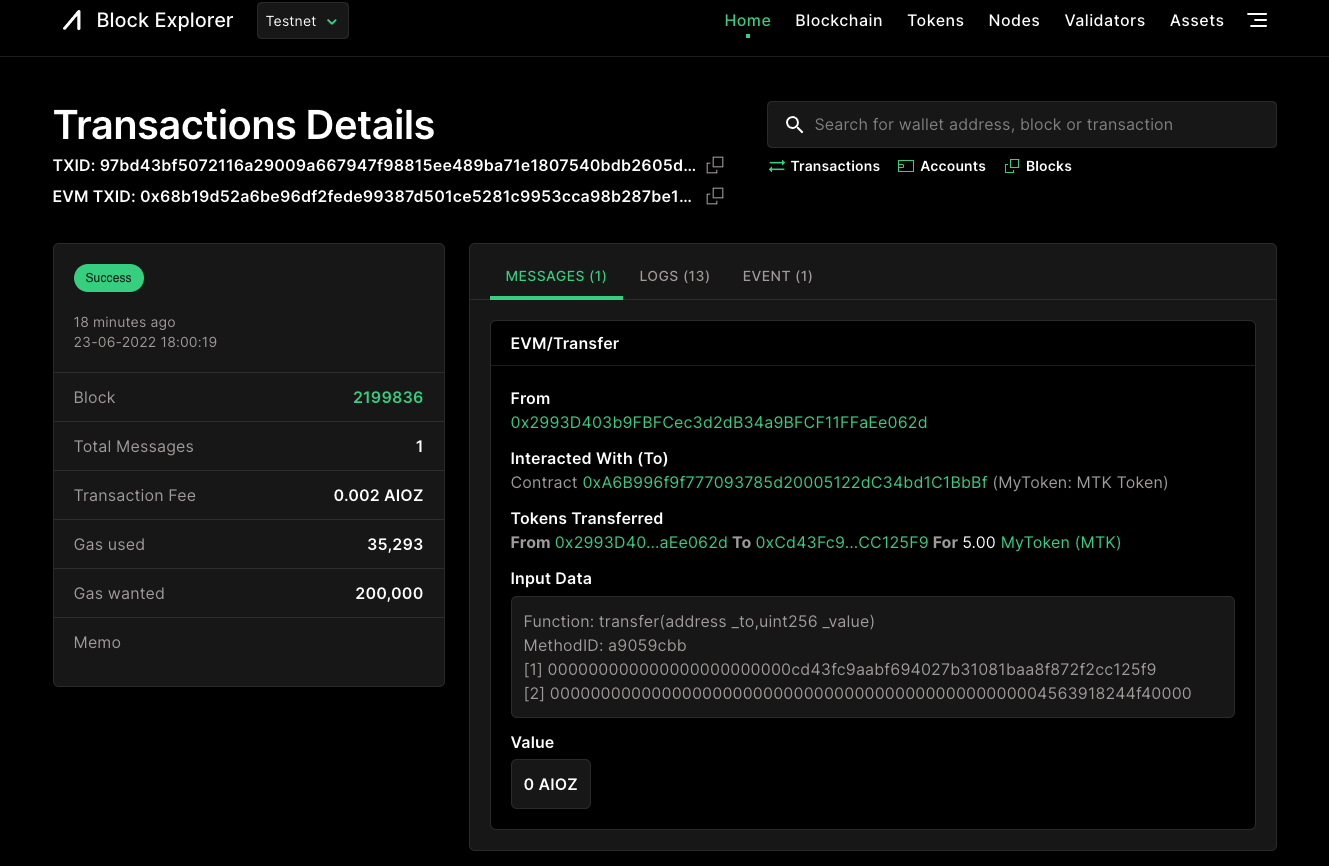