Remix: Deploy EVM Smart Contract
Remix (opens in a new tab) is an in-browser IDE for Solidity Smart Contracts. This guide will teach us how to deploy a contract to a running AIOZ Network through Remix and interact with it.
Set Up Development Environment
Connect Metamask to Deployed Account
Add AIOZ Network to Metamask
- Open the Metamask extension on your browser (you may have to log in to your Metamask account if you have not done so yet)
- Click on the top right circle and go to
Settings
>Networks
>Add Network
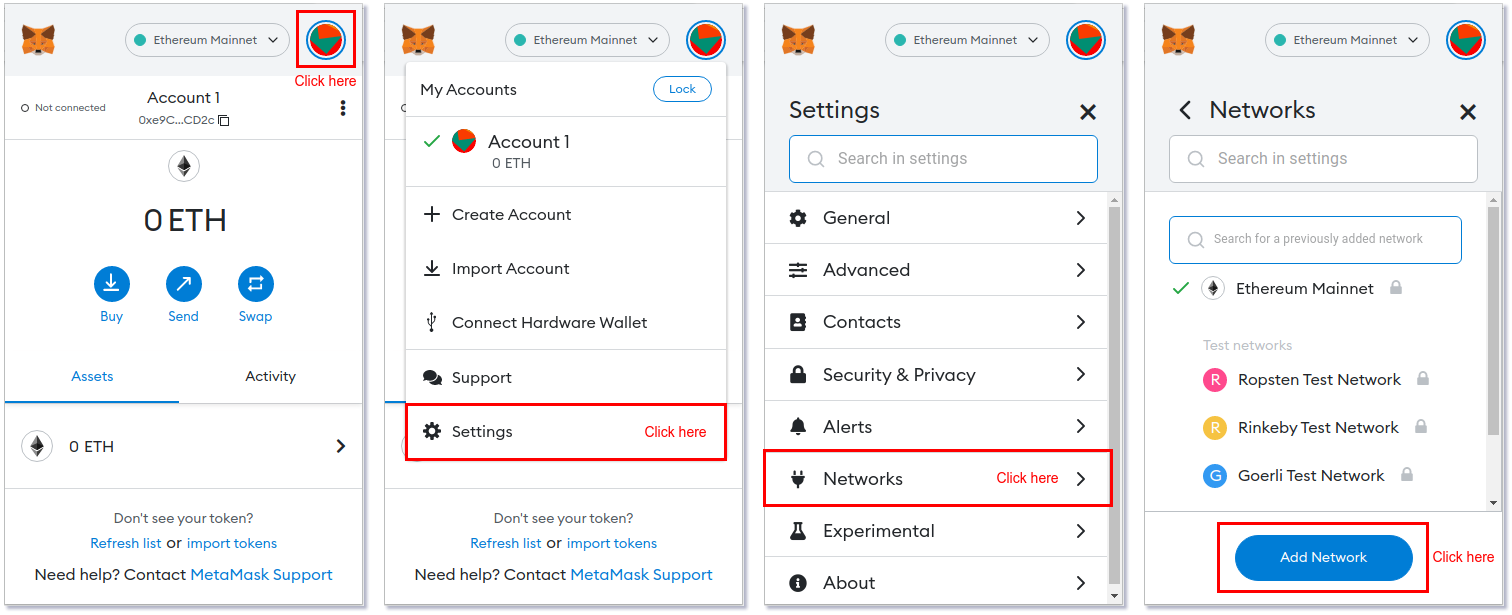
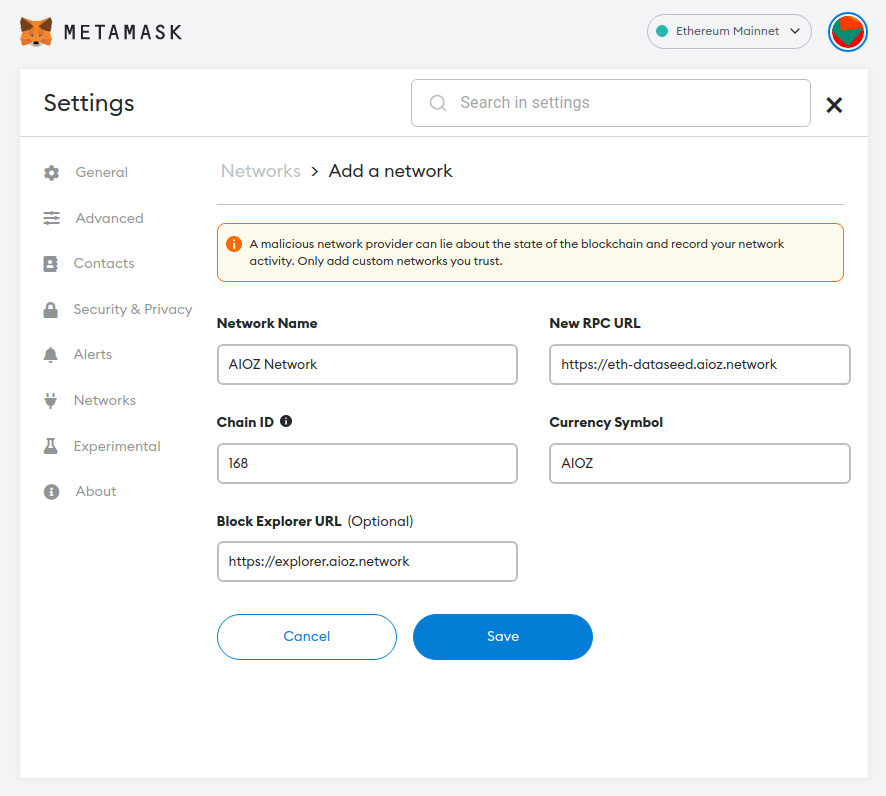
- To learn more how to add AIOZ Network in Metamask, please follow these articles:
Getting AIOZ Testnet from Faucet
- Access https://faucet-testnet.aioz.network (opens in a new tab)
- Enter wallet address to receive AIOZ testnet coin for gas fee to interact with AIOZ Blockchain Testnet
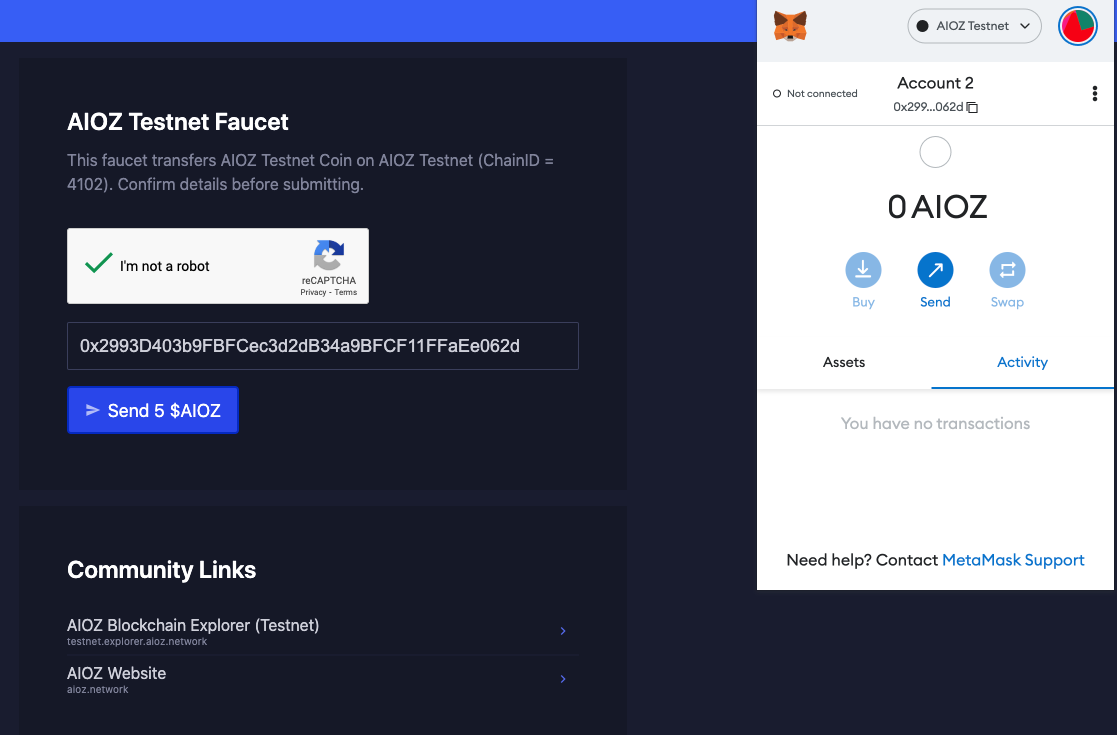
Writing Smart Contract
Go to Remix (opens in a new tab). There are some contracts in the File Explorer. Add a new file under source code to HelloAIOZ.sol
below.
// SPDX-License-Identifier: Unlicense
// Specifies that the source code is for a version of Solidity greater than 0.6.8
pragma solidity ^0.6.8;
contract HelloAIOZ {
// The public variables, which can be accessible from outside a contract
string public status;
constructor(string memory initStatus) public {
// setting `status` to initial value
status = initStatus;
}
// A publicly accessible function to updates `status`
function update(string memory newStatus) public {
status = newStatus;
}
}
This is the most basic smart contract on Solidity. The smart contract stores the status
message and lets everyone on the blockchain read/update status
.
Compile Smart Contract
On the left-most bar, select the Solidity Compiler
and compile the contract.
When the compilation process is completed without any errors or warnings, the contract is valid and ready to deploy.
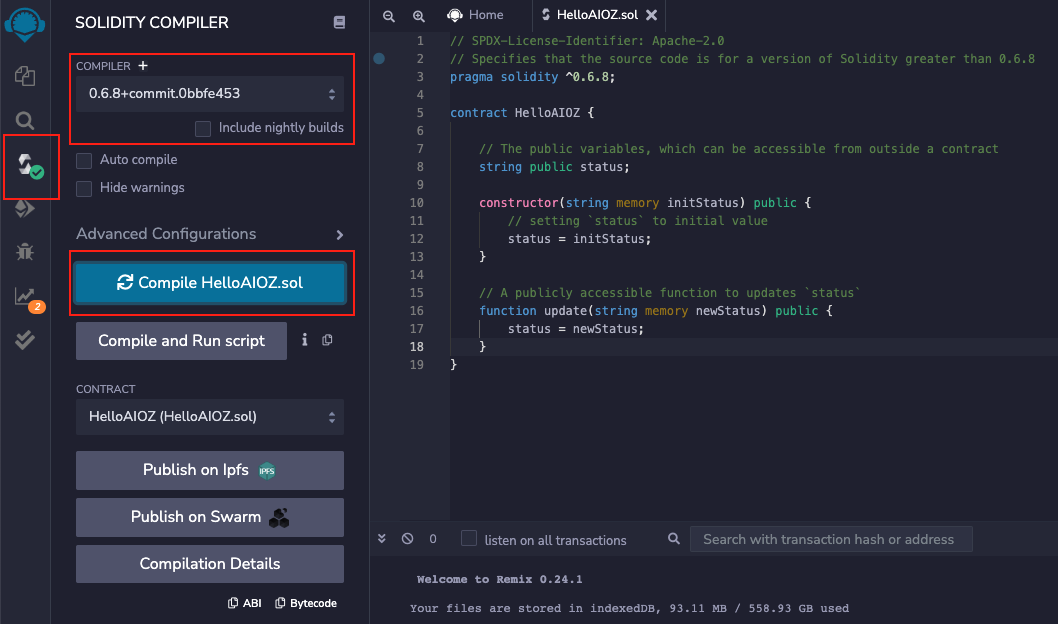
Deploying Smart Contract
On the left-most bar, select Deploy & Run transactions
- Select the account to deploy
- Select compiled smart contract to deploy
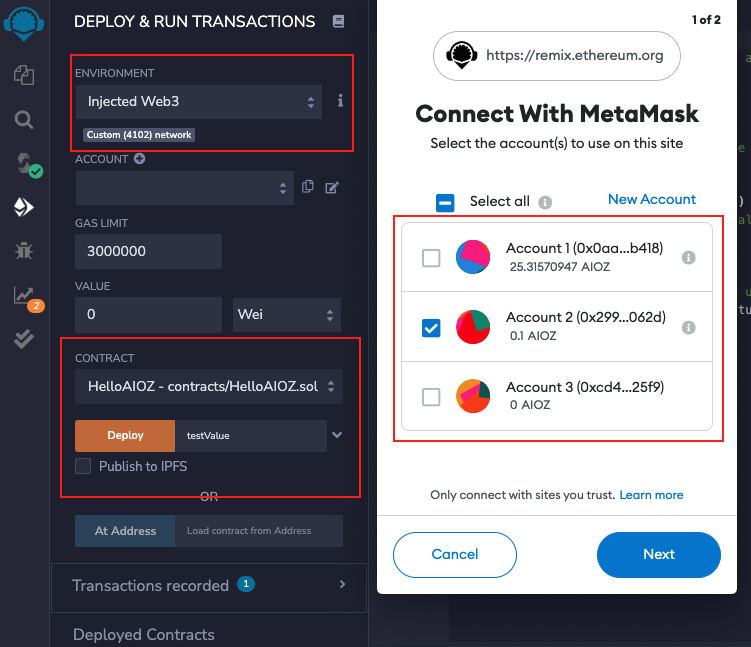
-
Enter initial value for
status
as parameter for contract initialized function -
Click
Deploy
>Confirm
Transaction on Metamask
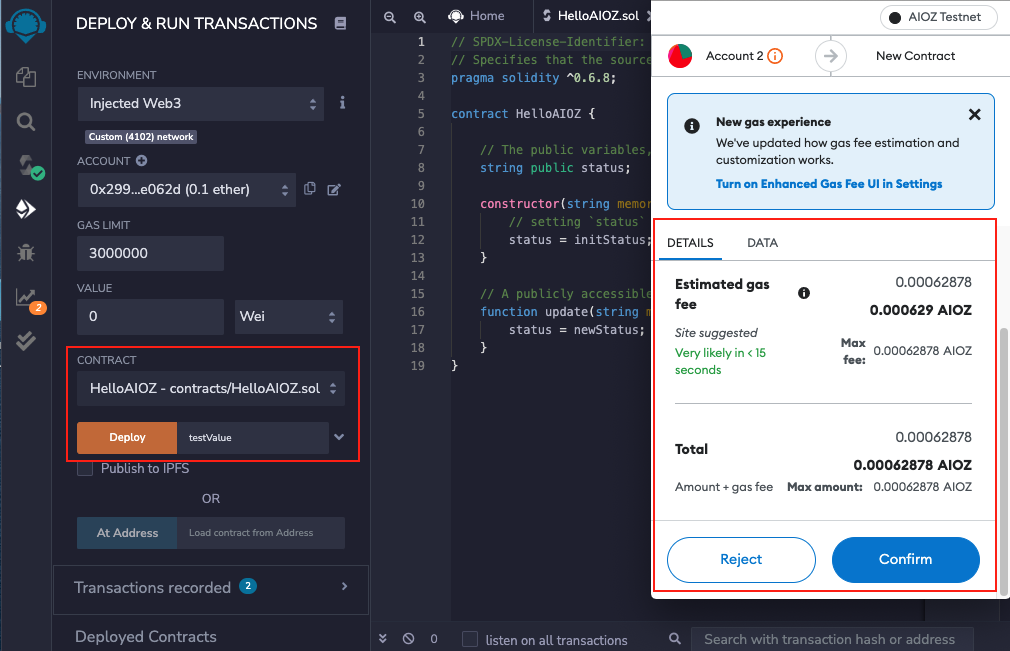
- Check Transaction Status from Remix Terminal
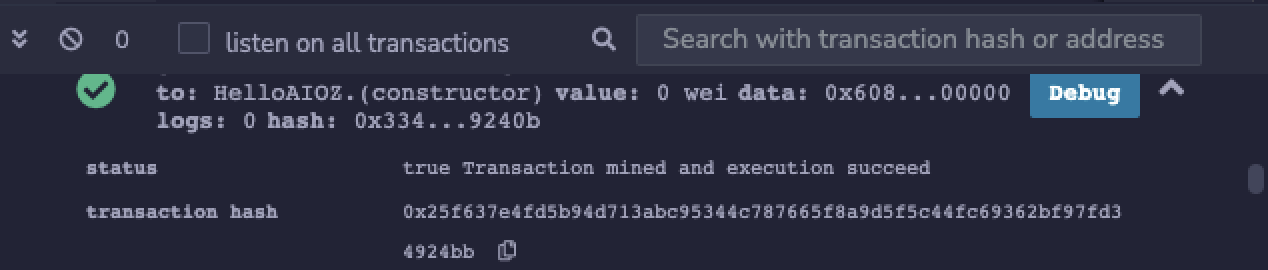
- Check Transaction Details with TX Hash
in AIOZ Blockchain Explorer (opens in a new tab)
Interact with Smart Contract
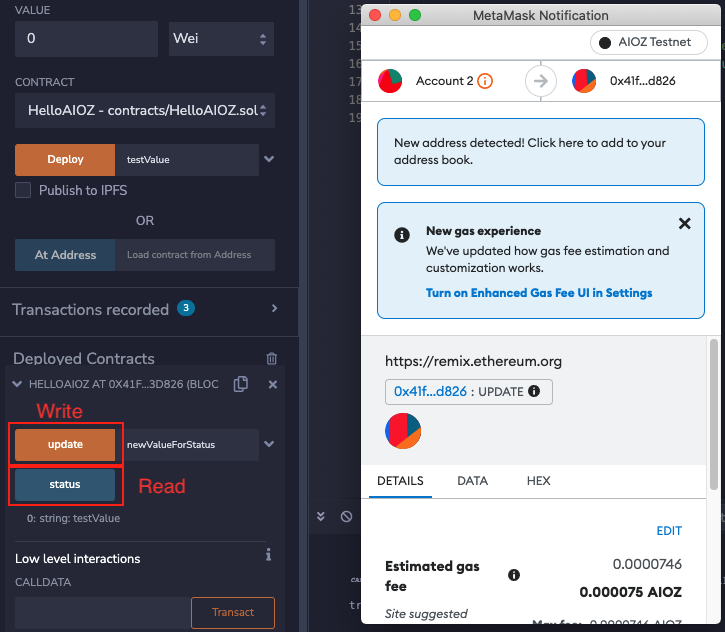
You can interact with all deployed contracts in Remix. Select deployed contract:
- Click
status
variable to read public value stored on blockchain - Click
update
> Enter new value > Metamask confirmation